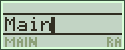
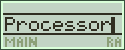
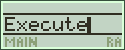
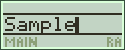
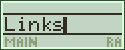
|
|
Assembly Language
This is not a comprehensive guide to Motorola 68k assembly
programming, but a definition of basic instructions that occur in many assembly programs. For a
comprehensive guide, check the
Motorola 68k Programmer's Reference Manual.
Addressing Modes
- Immediate (Constant) Data
- Immediate data is represented with a pound sign '#' followed by a number.
- Example: #2
-
- Data/Address Registers
- There are eight data registers and eight address registers, labelled zero
through seven. They are represented by either a 'd' or an 'a' followed by a number.
- Example: a0, d3
- Note: Register a7 is the stack pointer. Unless you moving things on and off of
the stack, do not use this register. Changing the stack pointer can cause the calculator
to crash.
-
- Indirect Register (Pointer)
- This refers to the data contained in the adress specified in an address register.
This is done by placing parentheses around the address register.
- Example: (a2)
- Post-decrement: To increment the data after fetching it, put a plus sign after
the right parenthesis: "(Ax)+".
- Pre-decrement: To decrement the data before fetching, put a minus sign before the
left parenthesis: "-(Ax)". Pre-decrement and post-decrement are most often used when
pushing and popping elements to and from the stack.
-
- Opcode Length Modifiers
- Adding ".b" to the end of an instruction will make the instruction read the data in bytes.
- Adding ".w" to the end of an instruction will cause the processor to treat the arguments
as words.
- Similarly, adding ".l" to the end of an instruction will treat the arguments as long words
(32 bits).
- Example: move.w #1,-(a7) ; Pushes the number 1 to
the stack as a word.
Basic Assembly Instructions
Note: Motorola processor instruction arguments generally follow the format source,
destination, which is reverse from Intel arguments.
- Movement Instructions
- move address, address - Moves the data in the source to the destination.
-
- movem registers, address - Moves the data in multiple locations to
adjacent memory locations, starting at address. Often used to push multiple
registers to the stack. Syntax for multiple registers is as follows: dx-dy/az-aw
(x<y and z<w).
- Also, movem address, registers does the reverse.
- Example: movem.l d3-d7/a2-a6,-(a7)
-
- clr address - Clears the contents at the specified register.
-
- lea address, An - "Load Effective Address" - loads the specified address to
the specified address register. Often used to change the stack pointer to allocate more
memory.
- Example: lea (a7,-3840),a7 ; Adds 3840 bytes to the
top of the stack.
-
- pea address - Pushes the data at the specified address to the stack.
-
- Arithmetic Instructions
- add Dx, address (or address, Dx) - Adds the source to the destination,
and places it in destination.
-
- addx Dx, Dy - Adds Dx to Dy, adds 1 if the extend flag is set, stores the
result in Dy.
-
- sub Dx, address (or address, Dx) - Subtracts the source from the
destination, and places the result in the destination
-
- neg address - Subtracts the data in address from 0.
-
- cmp address, address - Subtracts the source from the destination, but does
not store the result. Used to change the CCR.
-
- muls.w address, Dx - Multiplies the source by the destination, and places
the result in the destination. Both arguments are 16 bits, making the final output 32 bits.
-
- divs.w address, Dx - Divides the destination by the source, and places the
result in the destination. The quotient is placed in the lower word, and the remainder is
placed in the upper word.
-
- Branch Instructions
- bra #(immed) or address - Sets the Program Counter (PC) ahead by
a number of bytes equal to the argument.
- b(cc) #(immed) or address - Sets the PC forward by a number of bytes
equal to the argument if a certain condition is set. The branch condition is determined
by the one or two letters after 'b'. For instance, bpl will only jump forward if
the negative bit in the CCR is false.
- Condition Tests:
- cs - True if the Carry bit is set
- cc - True if the Carry bit is cleared
- eq - "Equal to Zero" - True if the Zero bit is set
- ne - "Not Equal to Zero" - True if the Zero bit is cleared
- vs - True if the Overflow bit is set
- vc - True if the Overflow bit is cleared
- mi - "Minus" - True if the Negative bit is set
- pl - "Plus" - True if the Negative bit is cleared
- ge - "Greater than or Equal to Zero" - True if the Negative and Overflow are
both cleared, or if they are both set.
- lt - "Less than or Equal to Zero" - True if the Negative bit is set and the
Overflow bit is cleared, or vice versa.
- gt - "Greater than Zero" - As ge, but the Zero bit must also be cleared.
- le - "Less than Zero" - As lt, but true if the Zero bit is set,
regardless of the other conditions.
-
- Rotate and Shift Instructions
- asl #(immed), Dx - "Arithmetic Shift Left" - Moves the Most Significant Bit
(MSB) into the Carry bit in the CCR, shifts each bit to the left, and inserts a 0 into the
Least Significant Bit (LSB).
-
- lsl #(immed), Dx - "Logical Shift Left" - Works exactly as asl.
-
- asr #(immed), Dx - "Arithmetic Shift Right" - Moves the LSB into the Carry bit
in the CCR, shifts each bit to the right, and inserts a copy of the old MSB to the new MSB.
-
- lsr #(immed), Dx - "Logical Shift Right" - As asl, but places a zero in
the MSB.
-
- rol #(immed), Dx - "Rotate Left" - Shifts each bit to the left, moves the
MSB to the Carry flag in the CCR, and moves the Carry flag into the LSB.
-
- roxl #(immed), Dx - "Rotate Left through Extend" - As rol, but the
Carry flag is then copied to the Extend bit.
-
- ror #(immed), Dx - "Rotate Right" - Shifts each bit to the right, moves the
LSB to the Carry flag in the CCR, and moves the Carry flag into the MSB.
-
- roxr #(immed), Dx - "Rotate Right through Extend" - As ror, but the
Carry flag is then copied to the Extend bit.
-
- swap Dx - Exchanges the high word in the specified register with the low word.
-
- Binary Logic
- not address - Flips all bits in the destination
-
- and Dx, address (or address, Dx) - Performs a bitwise AND (destination bit is true
only if both input bits are true) of the two values, and places the result in the
destination
-
- andi #(immed), address or Dx - As and, but the source is a constant
-
- or Dx, address (or address, Dx) - Performs a bitwise OR (destination bit is true if
either source bit is true) of the two values, and places the result in the destination
-
- ori #(immed), address or Dx - As or, but the source is a constant
-
- eor Dx, address (or address, Dx) - Performs a bitwise exclusive OR (destination bit is
true of one source bit is true and one is false) of the two values, and
places the result in the destination
-
- eori #(immed), address or Dx - As eor, but the source is a constant
|