Latest Updates
05-03-2011
Project and presentation are completed.
04-23-2011
During the past few days I have been struggling with threading and multi-processing. Something as simple as threading left and right turns to ensure we still have UI operating while the robot turns is a lot harder than it seems at first. First, when I start a new thread I need to know when to terminate it. If I simply “kill” the thread upon the stop button hit, I have absolutely no idea where I was in the thread, I loose touch with my current progress, I have no idea where exactly I terminated in the thread. I could rely strictly on my global buffer(how far I went so far), but I want a more graceful way of exiting the function than just to kill it half way, as I could kill it at the moment of me adding more data to the buffer. The second issue with just killing a thread is the problem with my Mutex lock variable. Once the Mutex is “locked” within a thread, only that thread can release it and no other. So, if I lock a thread out and then kill it before it has a chance to release mutex, I no longer have access to mutex, the best I can do is to simply wait until the system throws the “Abandoned mutex” error, and only then I can move on. Yet, another reason for a graceful exit. Third, I have created a global variable which is part of the right/left turn wait loop’s condition, so once the stop issues a “terminate = true” condition, the turning thread should see that and exit gracefully … however, again not that easy. After much research I found that C#’s compiler needs to have the keyword “volatile” declared before the variable for it to be seen in multiple threads(despite being declared globally), as for optimization purposes it(the compiler) only updates the variable in real-time in the main thread and not the child threads. Also, I discovered there is something called the system ThreadPool to which I can send functions and it can create a thread by itself(up to 25), while after 25 requests it puts others in the wait queue. While its nice to have such option, it also has its restrictions, for example: once I send a function to the ThreadPool, I can’t check the status of that particular thread, all I can know is when all in my working threads in the queue finish. This is not very useful as I can’t manually kill it if needs be and I have no info about a particular thread. Actually, I do have some knowledge of the threads inside the pool, by only through dynamically created pointers, thus, I can’t really use those in other functions in other threads as I wont know about them. And so here I am, struggling with a graceful termination, and Mutex cleanup on error of a thread. All of this work just to be able to stop the robot mid turn. Threading is definitely not easy, especially when working with the “robot”, GUI, TurnFunction threads all at the same time.
04-13-2011
After the last weeks meeting with Dr. Pankratz I was able to create easy conversions between high and low bytes to Int16 and backwards. This immensely helped in counting angle degrees and distance driven measurements. With these enhancements I was finally able to break up all of the scripts I previously used. Further, I did some research and figured out the proper use of the systems built in “Mutex” class. This made multi threading very easy and eliminated many side effects with which I have been struggling the whole past week. I also did a lot of research on delegates and multi-threading and finally got all of my cross threaded UI references to work correctly. I am now trying to go as fast as I can to implement my backtrack function. I believe I am very close, as I have a bump-check thread working(meaning I am now proficient with C# multi-threading) and all of my function calls to and from the robot are finally very generic and portable from one problem to the next.
04-06-2011
This week I have done a few changes to my existing code as recommended by Dr. Pankratz. First, I ensured, when the robot turns, that it turns about itself by making one wheel go forward and the other backwards instead of turning about one wheel. Second, I split up all of my scripts and instead of having the script “wait” for a certain turn angle while the robot turns before stopping, I do a while loop and constantly read the angle so as to allow other commands in the middle. I also made the following improvements: first, I started a new thread which constantly (every 15 milliseconds) check the bump sensor and ensure that its not bumped, if it is, it stops the robot, second, I consolidated the “read sensors” into one function. These improvements lead to a few new problems I am currently struggling with: first, if I consolidate the read sensor data into one function, than when I run the bump sensor thread all of my distance data gets “reset” since every “bump” read also reads the distance data(as the distance is collected between the data distance calls), second, for some odd reason, even if I separate my “bump check request” into its own function and just ask for bump sensor status and not distance, for some reason my distance data also gets reset. I have no idea why this is happening and I am still struggling with this problem, one solution is to collect distance when I check for bump, but that is a bit cumbersome…
03-29-2011
I am currently struggling with multi-processing issues in the project. When I send a script to the robot, it runs the script and does not respond until the script is complete. For example, I can send a script containing commands such as, drive forward x distance, when done stop. While the robot is driving (executing the script) it does not respond to any other commands, i.e. it does not listen for any more input and does not send feedback. My problem comes in when I try to send a script to the robot to turn 90 degrees and tell me the distance it turned. That is: start turning right, wait until the robot moved 90 degrees, stop the wheels, send the distance driven. This script alone works great. However, since my computer executes the algorithms so fast, it is always ahead of what the robot is doing, thus I have a hard time timing the serial port “read” command with the robot’s send distance command, to find out the angle the robot turned. If I check the serial ports “read” too soon, I might end up reading “junk”, if I do it too late, some other data might be in there and in the meantime I don’t want to pause the whole program(UI) and wait for the robot to finish turning. Multi-threading might be the key, but it makes the program a lot more sophisticated and difficult to follow and manage, so in the meantime I am thinking of a better design to do this in one thread and still be efficient(clever wait loops?).
3-21-2011
After the last meeting with Dr. Pankratz my project goal was slightly changed. I am now to focus more on the user interface and on the history log of a single robot’s activity. I implemented a speed “trackBar” to control the speed of the robot. I am further working on tracking the changes in speed and direction while the robot is moving. My next goal is to be able to “track” (with a stack) the slight turning of the robot while its moving; that is, if the robot is moving and the user slightly turns the robot with the turn buttons, I want to be able to track that deviation in order to be able to represent it with a stack.
Here is a screen shot of my UI so far:
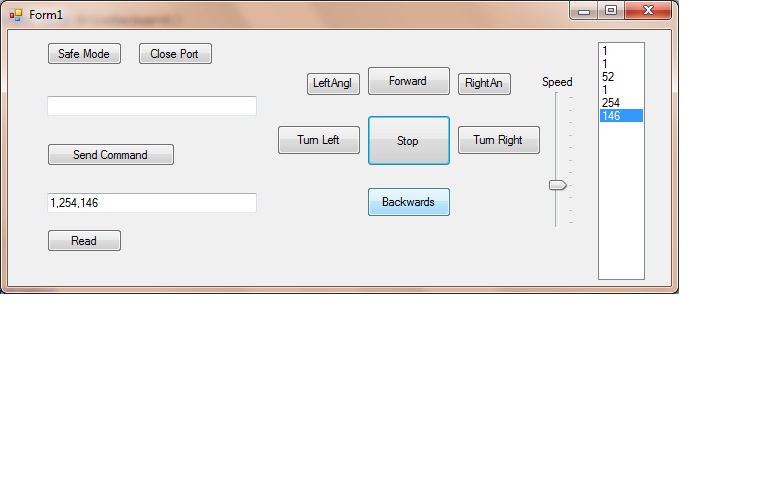
Here is a screen shot of my UI so far:
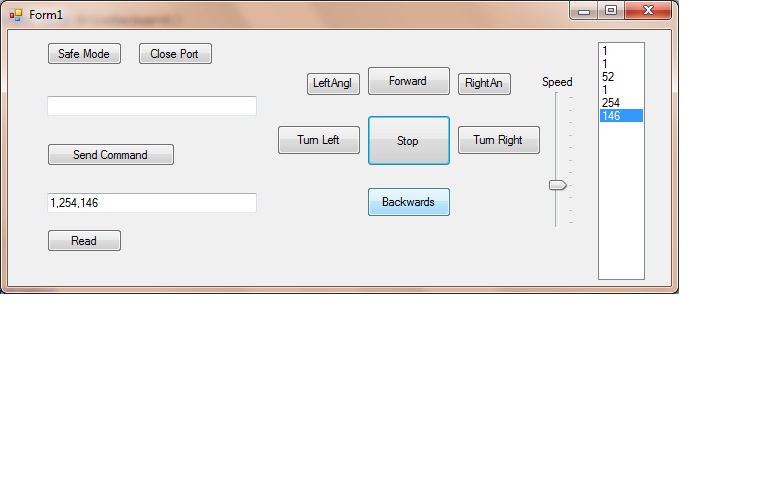
02-28-2011
I continued testing the robots throughout the week. I tested how accurately I can make them turn, the optimal speed for best distance reading and how to improve on my connection methods to make the robots more responsive. I also improved upon my screen, I added more buttons on the interface allowing me to easier test scripts, distances and response time. I also started saving different versions of my project to make my final presentation easier later on. The next step in my research is to further test and to implement samples of threading in C#. I spent quite a bit of time reading on “good practices in C# threading” and I implemented a few samples where my program can send signals on one thread, listen for freed back on the other while sending changes to the UI on the third. This is quite a bit more difficult than it may seem and I am still working on improving my current version. My next step is to improve and test threading to have a good base for starting communication algorithms between my robots. I am going to admit that I still have not done enough research on the GPS front. It just does not seem very promising and this point and I am focusing on making the robots work with a relative locating from a starting spot… I feel like I still need to keep braining storming for a better way to determine the location between the two robots… currently this is making me very uneasy as I still don’t even have a concrete conceptual idea on how to make that work…
02-21-2011
This week I was able to connect to both of the iRobots via Bluetooth under program control using C# (through the use of a serial port). The majority of this week went to testing the robots to make sure they still work. I also wrote a basic C# program and a small interface that I use to test the robots. In my interface I have a few buttons that can tell the robot to drive forward, backwards or to stop. I also tested a few “script” algorithms on the robots which made them drive in a square until I hit stop. Further, I tested the “distance” opcode (function) on the robot which returns the distance in cm that the robot has traveled since the last check. The function seems to represent data fast enough and accurately enough to be used in my project in keeping the relative distance between the two robots. I also continued the testing of the GPS. I discovered that the GPS works well(that is it sends accurate coordinates when moving AND standing still) if it gets the signal from at least 8 out of 10 satellites… the problem is… who knows that that depends on? Time of the day? Weather? My location on campus?... My next step is to determine if I can consistently get a good signal to read the location and use the GPS in my project.
02-10-2011
This week I Created Gantt chart to layout the goals which I want to achieve during the semester. I also acquired both iCreate robots and found all of the needed Bluetooth modules for them. I contacted Joel Rodriguez, who worked on the robots and Bluetooth last semester, and he showed me the code for how he connected to the robots using Bluetooth. Further, I went to the Create website and downloaded the manual for the robot with which I examined the instructions for connecting, sending and receiving commands to and from the robot using Bluetooth. During the last week I also connected the GPS via Bluetooth to my computer and got the initial connection code set up via C# sample code I got from Joel Rodriguez. My plans for the next week are expand on that sample to better interpret the information which the GPS returns. I also want to test the GPS unit to see how accurate it is in order to determine if it is precise enough or not for the purposes of my project.
02-01-2011
Created initial layout of the page.